Typescript Jupyter Book Template
Contents
Typescript Jupyter Book Template¶
This book uses typescript-jupyter-kernel to execute Typescript code.
const colors: string[] = ['#007acc', '#ffffff'];
// Either 0 or 1
const pixels: number[] = [0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,0,0,0,1,1,1,1,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,0,0,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,1,1,0,0,1,1,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,0,0,0,1,1,1,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,1,1,0,0,0,1,1,1,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0];
const logoContainer = document.createElement('div');
logoContainer.style = 'display: flex; flex-wrap: wrap; width: 125px; margin: auto;';
pixels.forEach(
(p, i) => {
const pixel = document.createElement('div');
pixel.style = `background: ${colors[p]}; border-radius: 4px; width: 4px; height: 4px; margin: 0 1px 1px 0;`;
logoContainer.appendChild(pixel);
}
);
document.body.appendChild(logoContainer);
jupyter.renderDom();
Basics¶
You can view console output:
console.log(
JSON.stringify(
{
hello: 'world'
},
null,
2
)
);
But it’s easier to read if you use the convenience jupyter
object:
jupyter.out(
JSON.stringify(
{
hello: 'world'
},
null,
2
)
);
{ "hello": "world" }
And you can render HTML on your Notebook!
jupyter.dom.window.document.body.innerHTML = `
<img src="https://media.giphy.com/media/VVgRNcBKp64NO/giphy.gif"/>
`;
jupyter.renderDom();
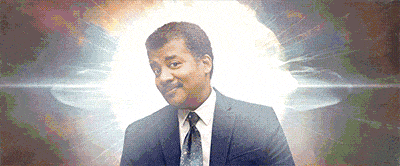
Note
For more complex examples visit the other pages in this book.
This is a small sample book to give you a feel for how book content is structured.
Jupyter Notebook is an integrated development environment (Web application) that allows you to edit and execute source codes and edit and share documents on a Web browser. (Taniguchi, 2017)
The previous citation follows the APA style, and the reference is
automatically generated from the references.bib
file (Mendeley is a great tool that was used to generate it).
The source structure of this book is as follows:
repo/
├─ book/
│ ├─ assets/
│ │ ├─ jeanlescure-logo.svg
│ ├─ notebook/
│ │ ├─ index.ipynb
│ ├─ index.md
│ ├─ _config.yml
│ ├─ _toc.yml
│ ├─ references.bib
├─ requirements.txt
├─ package.json
You can easily add equations using MathJax:
Tip
For more info visit: https://jupyterbook.org/content/math.html
The following bibliography also follows APA style and was generated automagically 🪄
Bibliograpy¶
- Taniguchi, 2017
Taniguchi, Y. (2017). Jupyter notebook. Kyokai Joho Imeji Zasshi/Journal of the Institute of Image Information and Television Engineers, 71, 240-243. doi:10.3169/itej.71.240